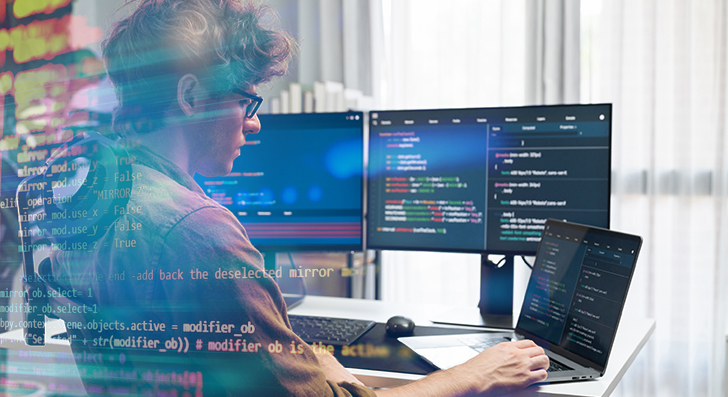
Scalability suggests your software can tackle advancement—additional end users, a lot more details, plus more website traffic—with no breaking. As being a developer, setting up with scalability in mind saves time and strain later on. Listed here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability is not something you bolt on later on—it should be section of the approach from the beginning. Lots of programs are unsuccessful after they mature speedy since the first design and style can’t cope with the extra load. Being a developer, you need to Consider early regarding how your method will behave stressed.
Commence by coming up with your architecture to become versatile. Avoid monolithic codebases in which anything is tightly connected. As an alternative, use modular design or microservices. These designs split your application into smaller, impartial sections. Each individual module or services can scale on its own devoid of affecting The full system.
Also, consider your databases from working day a person. Will it need to have to manage one million users or simply a hundred? Select the appropriate kind—relational or NoSQL—dependant on how your knowledge will grow. Prepare for sharding, indexing, and backups early, even if you don’t have to have them still.
Yet another crucial place is to stay away from hardcoding assumptions. Don’t write code that only functions below recent ailments. Give thought to what would materialize if your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that assistance scaling, like message queues or event-pushed techniques. These aid your app deal with much more requests with out getting overloaded.
When you build with scalability in your mind, you are not just making ready for fulfillment—you happen to be lowering potential headaches. A well-prepared process is simpler to maintain, adapt, and grow. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the ideal databases is actually a key Element of building scalable purposes. Not all databases are created the identical, and using the Erroneous one can gradual you down as well as result in failures as your application grows.
Start off by being familiar with your knowledge. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a superb in shape. They're strong with interactions, transactions, and consistency. In addition they assist scaling techniques like examine replicas, indexing, and partitioning to deal with extra targeted traffic and data.
When your information is more versatile—like person activity logs, product or service catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling huge volumes of unstructured or semi-structured data and might scale horizontally much more quickly.
Also, think about your examine and write designs. Are you presently performing numerous reads with much less writes? Use caching and read replicas. Are you presently handling a weighty generate load? Consider databases that could tackle higher compose throughput, or maybe event-primarily based knowledge storage methods like Apache Kafka (for short term facts streams).
It’s also good to think ahead. You may not need to have State-of-the-art scaling options now, but choosing a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your info dependant upon your entry designs. And constantly monitor database overall performance as you develop.
In brief, the proper database depends upon your app’s structure, velocity requires, And the way you anticipate it to develop. Consider time to pick sensibly—it’ll help you save loads of issues later on.
Enhance Code and Queries
Quickly code is key to scalability. As your app grows, each and every little delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s imperative that you Establish successful logic from the start.
Commence by creating clean, very simple code. Prevent repeating logic and remove something avoidable. Don’t select the most sophisticated solution if a straightforward one particular functions. Maintain your functions shorter, targeted, and easy to check. Use profiling resources to find bottlenecks—destinations in which your code takes far too extended to run or uses far too much memory.
Following, look at your databases queries. These usually gradual items down more than the code by itself. Make sure Just about every query only asks for the information you really have to have. Keep away from SELECT *, which fetches almost everything, and rather pick out particular fields. Use indexes to hurry up lookups. And stay clear of carrying out a lot of joins, Particularly across big tables.
When you notice precisely the same data getting asked for again and again, use caching. Retailer the final results quickly utilizing instruments like Redis or Memcached this means you don’t need to repeat expensive operations.
Also, batch your database functions after you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and will make your app much more productive.
Make sure to exam with large datasets. Code and queries that get the job done great with 100 records may well crash whenever they have to manage one million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when necessary. These ways help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and even more site visitors. If almost everything goes as a result of one server, it will rapidly become a bottleneck. That’s where load balancing and caching come in. Both of these instruments enable maintain your app quickly, stable, and scalable.
Load balancing spreads incoming visitors throughout numerous servers. Rather than 1 server doing all the do the job, the load balancer routes people to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can ship traffic to the others. Applications like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused rapidly. When users ask for exactly the same information yet again—like a product web site or maybe a profile—you don’t should fetch it from your databases anytime. It is possible to serve it within the cache.
There are 2 common different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
two. Shopper-side caching (like browser caching or CDN caching) outlets static information near the user.
Caching lowers database load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust often. And usually make certain your cache is up-to-date when data does adjust.
In short, load balancing and caching are basic but impressive resources. Jointly, they assist your app take care of more consumers, keep fast, and Get well from difficulties. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable programs, you require tools that let your app increase quickly. That’s where cloud platforms and containers come in. They provide you overall flexibility, lower setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you will need them. You don’t must get components or guess long run potential. When targeted visitors improves, you can add much more assets with just a couple clicks or routinely working with car-scaling. When website traffic drops, you may scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection instruments. It is possible to target constructing your app rather than handling infrastructure.
Containers are another vital Device. A container packages your app and all the things it really should operate—code, libraries, options—into 1 device. This causes it to be straightforward to move your application amongst environments, out of your laptop into the cloud, without the need of surprises. Docker is the most well-liked Device for this.
Once your app uses various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your app into expert services. You'll be able to update or scale parts independently, and that is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment usually means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you would like your application to mature with no restrictions, begin working with these resources early. They help you save time, minimize possibility, and assist you to keep centered on developing, not repairing.
Observe Every thing
When website you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is carrying out, place difficulties early, and make better selections as your application grows. It’s a vital A part of creating scalable devices.
Get started by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—monitor your app as well. Keep watch over just how long it's going to take for users to load pages, how often mistakes occur, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential difficulties. As an example, Should your response time goes above a Restrict or simply a company goes down, you'll want to get notified promptly. This can help you correct challenges speedy, generally ahead of consumers even recognize.
Monitoring is usually handy if you make adjustments. Should you deploy a fresh characteristic and see a spike in glitches or slowdowns, it is possible to roll it back before it results in authentic injury.
As your app grows, website traffic and knowledge improve. Without the need of monitoring, you’ll miss out on signs of hassle right up until it’s as well late. But with the right instruments in place, you keep in control.
To put it briefly, monitoring allows you maintain your application trustworthy and scalable. It’s not just about spotting failures—it’s about understanding your process and ensuring it really works properly, even stressed.
Ultimate Views
Scalability isn’t just for major organizations. Even compact applications require a robust foundation. By planning carefully, optimizing correctly, and utilizing the correct instruments, you are able to Make applications that expand effortlessly with out breaking stressed. Get started little, Consider big, and Construct clever.